The plugin Upload Modal can be integrated in your code and make it possible to upload Vimeo videos from anywhere. This is step by step guide.
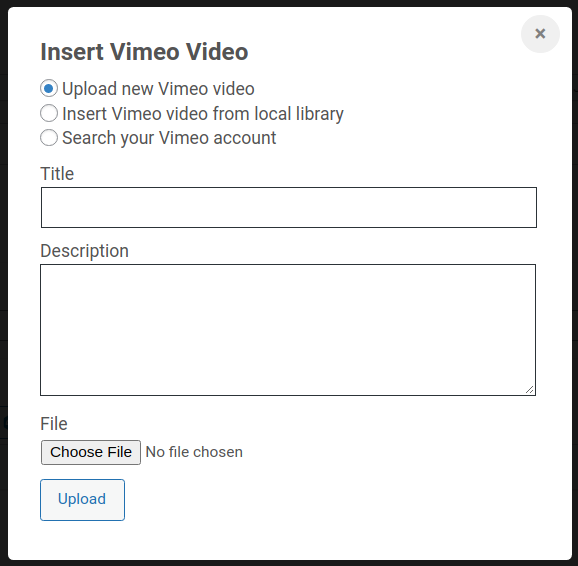
1. Enqueue the required JS
This steps outlines how to enqueue the required javascript files that will be used to enqueue all of your functionality. Let’s call your file custom-vimeo.js.
In functions.php use this code, but make sure you set correct file paths.
<?php
/**
* Enqueue the upload modal
*/
function dgv_823828_enqueue_scripts() {
// Check where you want to initialize this functionality
// ... other conditions can be here or none at all.
if(is_page(1111)) {
wp_enqueue_style('dgv-upload-modal');
wp_enqueue_script('dgv-upload-modal');
wp_enqueue_script(
'your-custom-script',
'https://url-to-script/custom-vimeo.js',
['jquery', 'dgv-upload-modal'],
null,
true
);
}
}
add_action('wp_enqueue_scripts', 'dgv_823828_enqueue_scripts');
2. Content in your template file (modal trigger) and results
All you need is to have two elements, a modal trigger for input and results output.
The modal trigger can be a button, link or something else that will trigger the modal.
<button id="your-upload-button">Upload Vimeo</button>
Results can be printed in specific element on your page as follows.
<div id="your-results-element"></div>
3. Content in custom-vimeo.js
This is an example code of handling the events that are triggered once a video is uploaded successfully.
(function ($) {
// Unique id of the form, used to check if you are
// targeting the right event.
var BUTTON_CONTEXT = 'your_unique_events_namespace';
// This is the value obtained from the modal.
// Use this function to save this value somewhere...
// In the example we are just printing it in a paragraph...
var load_value = function (uri, context) {
if(BUTTON_CONTEXT === context) {
$('#your-results-element').text(uri);
}
}
// Trigger the upload modal on button click
$(document).on('click', '#your-upload-button', function (e) {
e.preventDefault();
var uploadModal = new WPVimeoVideos.UploaderModal(BUTTON_CONTEXT);
uploadModal.open();
});
// Handle the insert result.
$(window).on('wpdgv.events.insert', function (e, data) {
load_value(data.uri, data.context);
});
// Hanlde the upload result.
$(window).on('wpdgv.events.upload', function (e, data) {
load_value(data.uri, data.context);
});
})(jQuery);